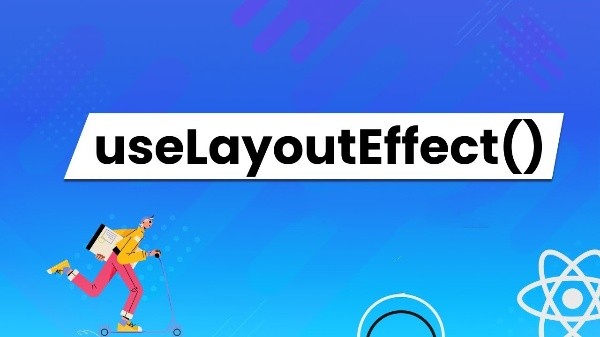
Mastering React: Unlock the Power of useLayoutEffect for Precise Visual Effects
React is a powerful JavaScript library for building user interfaces. One of the key features of React is its ability to efficiently update and render components. In this post, we’re going to take a look at the useLayoutEffect hook, which allows us to synchronously update a component’s layout and paint. First, let’s start with a basic example. Suppose we have a simple component that displays a counter:
import { useState, useLayoutEffect } from 'react'
function Counter() {
const [count, setCount] = useState(0);
useLayoutEffect(() => {
document.title = `Count: ${count}`;
}, [count]);
return (
<>
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</>);
};
In this example, we’re using the useLayoutEffect hook to update the document’s title whenever the count state changes. The first argument to useLayoutEffect is a callback function that will be executed after the layout and paint have been updated. The second argument is an array of dependencies, which tells React when to re-run the effect. In this case, we’re only re-running the effect when the count state changes.
The useLayoutEffect hook works similarly to the useEffect hook, but with one important difference: useLayoutEffect runs synchronously after the layout and paint have been updated, whereas useEffect runs asynchronously. This means that useLayoutEffect can be used to perform layout and paint updates that need to be synchronous. This is particularly useful when working with animations or other visual effects that require precise timing.
In conclusion, useLayoutEffect hook is a powerful tool that allows you to synchronously update a component’s layout and paint. It’s particularly useful when working with animations or other visual effects that require precise timing. It’s also worth noting that useLayoutEffect can cause layout thrashing if not used properly. Layout thrashing happens when multiple layout updates are triggered in quick succession, causing the browser to constantly recalculate the layout and paint. To avoid this, it’s important to make sure that the effect only updates the layout and paint when absolutely necessary.
Another thing to keep in mind is that useLayoutEffect can have an impact on the performance of your application, especially on low-end devices or slow networks. So, it’s important to use it judiciously and only when it’s truly necessary.
To sum up, useLayoutEffect is a powerful tool that allows you to synchronously update a component’s layout and paint. It’s particularly useful when working with animations or other visual effects that require precise timing. However, it can cause layout thrashing if not used properly, and it can also have an impact on the performance of your application. So, use it judiciously and only when it’s truly necessary.
I hope this post has helped you understand how to use useLayoutEffect in your React projects. If you have any questions or feedback, please feel free to leave a comment below. Happy coding!